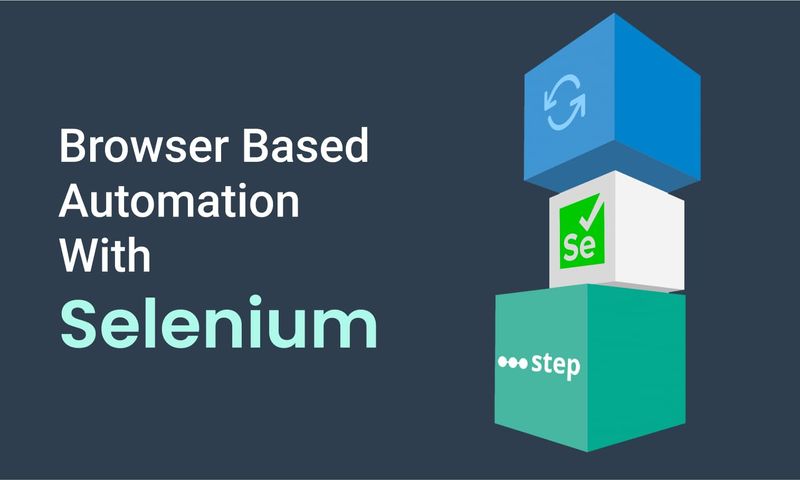
Mastering Browser Automation with Selenium: 10 Powerful Use Cases and Concepts
Introduction to Browser Automation
Browser automation refers to the practice of using software tools to programmatically control web browsers. This is typically done to perform repetitive tasks, conduct testing, or scrape web data without human intervention. One of the most popular tools for browser automation is Selenium, an open-source framework that supports multiple programming languages, including Python.
What is Selenium?
Selenium is a powerful tool suite designed for browser automation. It provides interfaces to create robust, browser-based regression automation suites and tests. Selenium supports multiple browsers (Chrome, Firefox, Safari, and Edge) and can be integrated with various testing frameworks.
Key Components of Selenium
Selenium is composed of several key components that together provide a comprehensive suite for browser automation. Each component serves a specific purpose and can be used independently or in conjunction with others to meet various automation needs.
Selenium WebDriver
Selenium WebDriver is the core component of Selenium, responsible for driving the browser and interacting with web elements. It provides a programming interface to create and execute test scripts, enabling direct communication with the web browser. WebDriver supports a wide range of browsers, including Chrome, Firefox, Safari, and Edge, making it a versatile tool for cross-browser testing.
Use Cases:
- Functional Testing: WebDriver is used to perform functional tests by simulating user interactions like clicking buttons, entering text, and navigating through web pages. This helps ensure that web applications behave as expected.
- End-to-End Testing: It is employed to test complete workflows from start to finish, validating the entire application process, such as logging in, performing transactions, and logging out.
- Data Extraction: WebDriver can be used for web scraping tasks where dynamic content loaded via JavaScript needs to be captured and processed.
Selenium IDE
Selenium IDE (Integrated Development Environment) is a browser extension that allows users to record, edit, and playback Selenium test cases. It is designed for simplicity and ease of use, making it accessible for users who may not have extensive programming knowledge. Selenium IDE supports the creation of test scripts by recording user actions on a web page, which can then be played back to perform automated tests.
Use Cases:
- Rapid Test Development: Selenium IDE is ideal for quickly developing test cases without writing code. Users can record their interactions with the web application and convert them into test scripts.
- Prototyping: It can be used to create prototypes of test cases to demonstrate and validate test scenarios before developing more complex test scripts with WebDriver.
- Training and Demonstration: Selenium IDE serves as a valuable tool for training new testers on the basics of test automation and for demonstrating test automation concepts to stakeholders.
Selenium Grid
Selenium Grid is a tool that allows running Selenium tests on multiple machines and browsers simultaneously, facilitating parallel execution and browser automation. This capability is essential for large-scale test environments where tests need to be executed across various browser and OS combinations to ensure comprehensive coverage.
Use Cases:
- Cross-Browser Testing: Selenium Grid enables testing across different browsers (e.g., Chrome, Firefox, Safari) and browser versions, ensuring that the web application works correctly regardless of the user’s browser choice.
- Parallel Execution: By running tests in parallel, Selenium Grid significantly reduces the time required to execute a large suite of tests, making the testing process more efficient.
- Distributed Testing: Selenium Grid supports distributed test execution by running tests on remote machines, which can be beneficial for teams with distributed infrastructure or cloud-based test environments.
Integration and Combined Use
The key components of Selenium—WebDriver, IDE, and Grid—can be integrated to create a robust testing framework. For example, testers might use Selenium IDE to quickly create and debug test scripts, then export these scripts to WebDriver for more advanced testing scenarios. Selenium Grid can then be employed to execute these tests across multiple environments simultaneously, ensuring comprehensive test coverage and efficient execution.
Generic Examples:
- WebDriver and Grid: A company developing a web application could use WebDriver to create detailed test scripts that interact with all aspects of the application. These scripts can then be run on a Selenium Grid setup to test the application across different browsers and operating systems simultaneously, ensuring compatibility and performance under various conditions.
- IDE and WebDriver: A team might use Selenium IDE to record basic test scripts during the initial phase of test development. These scripts can later be refined and expanded using WebDriver to include more complex logic and validations. This approach allows for rapid prototyping and iterative development of test cases.
- End-to-End Solution: In a comprehensive testing strategy, an organization could use Selenium IDE for quick recording and debugging of test cases, WebDriver for developing robust and detailed test scripts, and Selenium Grid for executing these tests across multiple environments. This combined use ensures that the application is thoroughly tested for functionality, compatibility, and performance.
Setting Up Selenium with Python
To use Selenium with Python, you need to install the Selenium package and a web driver for the browser you intend to automate (e.g., ChromeDriver for Chrome). Here are the steps to install and setup Selenium:
How to use browser automation to open a website using python with selenium in Chrome browser
- In order to install python on your device, you can use the official website: https://www.python.org/downloads/. You can download the latest version for windows/linux/mac.
- To install Selenium with Python, follow the instructions given at Selenium, Python Package Index (PyPI).
- If you have pip (package installer for Python) on your system, you can simply install or upgrade the Python bindings.You can run the following from a command prompt. If you are using Visual Studio Code, it is best to run this from within VS Code (from Terminal under the View menu):
pip install -U selenium.
- Selenium requires a driver to interface with the chosen browser. Chrome, for example, requires chromedriver, which needs to be installed before any tests can be run. This can be installed from https://chromedriver.chromium.org/downloads Website. The driver should be installed according to the Browser’s version and device type (Mac/Windows/Linux). Make sure it’s in your PATH, e. g., place it in “C:\chromedriver.exe”
- Failure to add the driver will give you an error selenium.common.exceptions.WebDriverException: Message: ‘chromedriver’ executable needs to be in PATH. Other supported browsers will have their own drivers available.
- After the installation and setup of selenium and webdrivers, You can start writing code to use browser automation in the chosen browser.
from selenium import webdriver
# Specify the path to the chromedriver executable
driver = webdriver.Chrome(executable_path=‘/path/to/chromedriver’)
# Open a website
driver.get(‘https://www.example.com’)
# Perform actions on web elements
element = driver.find_element_by_name(‘q’)
element.send_keys(‘Selenium Python’)
element.submit()
# Close the browser
driver.quit()
Conceptual Uses of Selenium
Web Testing:
Automated testing with Selenium is one of its primary use cases. Selenium enables testers to simulate user interactions with a web application, including clicking buttons, entering text, and navigating through different pages. This simulation is essential for regression testing, where the objective is to ensure that new code changes do not introduce bugs into existing functionalities.
1.End-to-End Testing:
Selenium can be used to perform comprehensive end-to-end tests that mimic real user behavior. This includes logging into the application, performing various actions like creating or deleting records, and verifying the outcomes. Such tests help in validating the entire workflow of an application from start to finish.
2. Cross-Browser Testing:
Selenium supports multiple browsers such as Chrome, Firefox, Safari, and Edge. This capability is crucial for cross-browser testing, ensuring that web applications function correctly across different browsers.
3. Integration with CI/CD Pipelines:
Selenium tests can be integrated into Continuous Integration/Continuous Deployment (CI/CD) pipelines using tools like Jenkins, GitLab CI, or Travis CI. This ensures automated execution of tests whenever there is a code change, providing immediate feedback to developers.
Example:
pipeline {agent anystages {stage(‘Build’) {steps {sh ‘pip install selenium’}}stage(‘Test’) {steps {sh ‘python run_tests.py’}}}}
Web Scraping:
Selenium is particularly useful for web scraping tasks involving websites that load content dynamically using JavaScript. Traditional scraping tools, such as BeautifulSoup and Scrapy, operate on the static HTML content of web pages and might struggle with such sites. Selenium, however, can render the JavaScript content, making it possible to extract data from complex, dynamic web pages.
1. Extracting Data from Dynamic Web Pages:
Many modern websites use JavaScript frameworks to load content asynchronously. Selenium can wait for these elements to load and then extract the required information.
2. Navigating Through Pagination:
Many websites display data across multiple pages. Selenium can handle pagination by clicking through each page and scraping the required data.
Automating Repetitive Tasks
Selenium excels at automating repetitive tasks that would otherwise be time-consuming and prone to human error. These tasks include filling out web forms, downloading files, and performing routine checks.
1.Filling Out Web Forms:
Automating the process of filling out web forms can save a significant amount of time, especially when dealing with large datasets or repetitive entries.
2. Downloading Files:
Automating file downloads from the web can streamline workflows that require regularly fetching data or reports.
3. Routine Checks and Monitoring:
Selenium can be used to perform routine checks, such as verifying the availability of a website or monitoring specific elements on a page.
Advanced Selenium Concepts
- Handling Pop-ups and Alerts: Selenium can manage browser pop-ups and JavaScript alerts using the Alert interface.
- Working with Multiple Windows: Selenium can switch between multiple browser windows or tabs using window handles.
- Explicit and Implicit Waits: Selenium offers different waiting mechanisms to handle elements that take time to load.
- Taking Screenshots: Selenium can capture screenshots of web pages for documentation or debugging purposes.
Conclusion
Selenium is a versatile and powerful tool for browser automation, capable of handling a wide range of tasks from web testing to scraping dynamic content. By leveraging Selenium with Python, developers and testers can enhance their productivity, ensure the robustness of web applications, and automate repetitive web-based tasks effectively.